Exterminate!
| Anke (encarsia) | Also available in: Deutsch
The VTE terminal widget
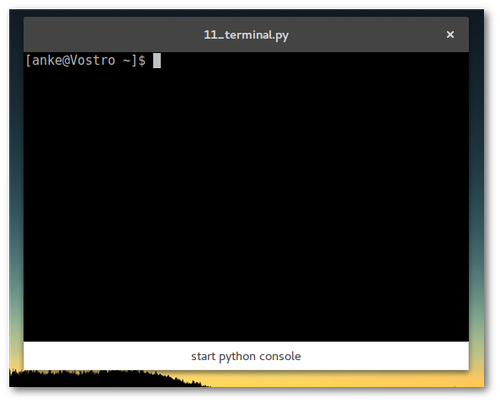
Glade
The widget can be found in the lower part of the widget side bar and provides a complete terminal emulator. To close the window on the exit
command the child-exited signal has to be assigned.
A button click shall open a Python prompt within the terminal window, so we need the familiar clicked signal.
Python
Elements used in Glade that are not part of the Gtk module have to be registered as a GObject object (this is also required when using a GtkSourceView widget as the functionality is provided by the GtkSource module):
GObject.type_register(Vte.Terminal)
The terminal emulator is initiated by calling spawn_sync
expecting 7 parameters. Detailed information on the parameters are available in the documentation but for a common start a lot of defaults and Nones will do:
terminal.spawn_sync( Vte.PtyFlags.DEFAULT, None, ["/bin/bash"], None, GLib.SpawnFlags.DEFAULT, None, None, )
The feed_child
function must be called to send a command to the console. The expected parameters are the string including a newline and the length of the string:
command = "python\n" x.terminal.feed_child(command,len(command))
Listings
Glade
<?xml version="1.0" encoding="UTF-8"?> <!-- Generated with glade 3.20.0 --> <interface> <requires lib="gtk+" version="3.20"/> <requires lib="vte-2.91" version="0.46"/> <object class="GtkApplicationWindow" id="window"> <property name="can_focus">False</property> <signal name="destroy" handler="on_window_destroy" swapped="no"/> <child> <object class="GtkBox"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="orientation">vertical</property> <child> <object class="VteTerminal" id="term"> <property name="visible">True</property> <property name="can_focus">True</property> <property name="has_focus">True</property> <property name="hscroll_policy">natural</property> <property name="vscroll_policy">natural</property> <property name="encoding">UTF-8</property> <property name="scroll_on_keystroke">True</property> <property name="scroll_on_output">False</property> <signal name="child-exited" handler="on_window_destroy" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">0</property> </packing> </child> <child> <object class="GtkButton" id="button"> <property name="label" translatable="yes">start python console</property> <property name="visible">True</property> <property name="can_focus">True</property> <property name="receives_default">True</property> <signal name="clicked" handler="on_button_clicked" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">False</property> <property name="position">2</property> </packing> </child> </object> </child> <child type="titlebar"> <placeholder/> </child> </object> </interface>
Python
#!/usr/bin/python # -*- coding: utf-8 -*- import os import gi gi.require_version("Gtk", "3.0") gi.require_version("Vte", "2.91") from gi.repository import Gtk, Vte, GObject, GLib class Handler: def on_window_destroy(self, *args): Gtk.main_quit() def on_button_clicked(self, widget): command = "python\n" x.terminal.feed_child(command.encode()) class Example: def __init__(self): self.builder = Gtk.Builder() GObject.type_register(Vte.Terminal) self.builder.add_from_file("11_terminal.glade") self.builder.connect_signals(Handler()) self.terminal = self.builder.get_object("term") self.terminal.spawn_sync( Vte.PtyFlags.DEFAULT, None, ["/bin/bash"], None, GLib.SpawnFlags.DEFAULT, ) window = self.builder.get_object("window") window.show_all() def main(self): Gtk.main() x = Example() x.main()
Comments
Comments powered by Disqus