View from the window
| Anke (encarsia) | Also available in: Deutsch
Minimal example
Glade
After launching Glade the application view is divided in 3 areas: window/widget selection on the left, the project view in the centre and a tree/property (including signal) view of project elements on the right.
Now a window is created and an ID assigned to be able to address the element in the source code.
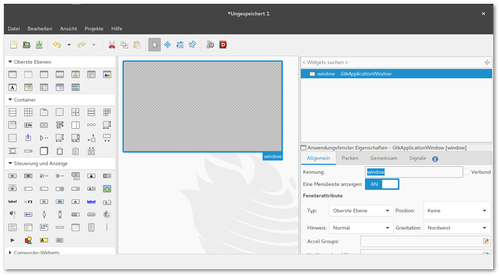
To call functions from widgets they have to be connected to signals. Depending on the kind of graphical object signals can be emitted by clicking, marking, editing, switching etc.
If the example window should be able to be closed on clicking the [X] button the signal destroy is required. The entry field to specify the function provides a convenient suggestion function to reduce key input following the pattern on_id_signal
.
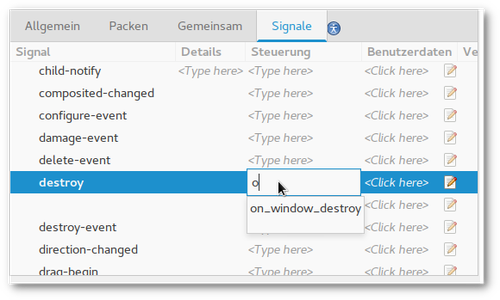
Glade does not generate GTK+ source code but a XML formatted file accessible to GtkBuilder (see listing below).
Python
First things first. GtkBuilder is provided by the Gtk module from the Python GObject Introspection bindings:
import gi gi.require_version('Gtk','3.0') from gi.repository import Gtk
After initing Gtk.Builder()
the Glade file(s) are added.
builder.add_from_file(gladefile)
It may be convenient to work with multiple files in one project. In this case you have to bear in mind that if there are elements with the same identifier name only the element of the last loaded file can be addressed by get_object(id)
.
Second step is connecting the signals. It comes in handy to store these functions in an own class:
self.builder.connect_signals(Handler())
So this basic example script opens an empty window hat can be closed on clicking the close button.
Ohne Glade
This example corresponds to the basic example of the Python GTK+ 3 tutorial:
import gi gi.require_version('Gtk', '3.0') from gi.repository import Gtk win = Gtk.Window() win.connect("delete-event", Gtk.main_quit) win.show_all() Gtk.main()
The definded window does not contain any elements like boxes, buttons, bars, menus and other widgets.
Listings
Glade
<?xml version="1.0" encoding="UTF-8"?> <!-- Generated with glade 3.20.0 --> <interface> <requires lib="gtk+" version="3.20"/> <object class="GtkApplicationWindow" id="window"> <property name="can_focus">False</property> <property name="title" translatable="yes">Titel</property> <signal name="destroy" handler="on_window_destroy" swapped="no"/> <child> <placeholder/> </child> <child> <placeholder/> </child> </object> </interface>
Python
#!/usr/bin/python # -*- coding: utf-8 -*- import gi gi.require_version("Gtk", "3.0") from gi.repository import Gtk class Handler: def on_window_destroy(self, *args): Gtk.main_quit() class Example: def __init__(self): self.builder = Gtk.Builder() self.builder.add_from_file("01_minimal.glade") self.builder.connect_signals(Handler()) window = self.builder.get_object("window") window.show_all() def main(self): Gtk.main() x = Example() x.main()
Comments
Comments powered by Disqus