Push the button
| Anke (encarsia) | Also available in: Deutsch
Buttons and labels
Glade
Based on the first example some elements are added, a label, a button and a togglebutton. Any control or display widget requires a container. In this example a vertically arranged container box is created. Boxes can be extended, downsized, rearranged and contain further container widgets.
Now the clicked and toggled signal are assigned to the button and togglebutton element. The label widget's purpose ist to display text so there is no signal assignment required.
Glade provides a preview function to test if the correct reaction is triggered.
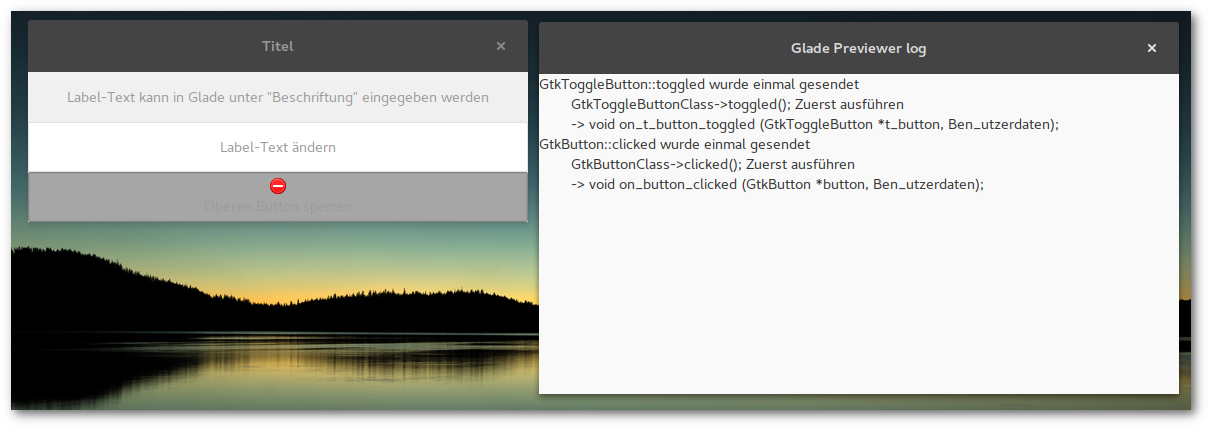
Python
On a button click the label widget should change the diplayed text randomly chosen from a given list.
All Gtk.Builder elements can be addressed by the get_object
function.
Gtk.Builder.get_object("name").function(options) #Beispiel GtkLabel Gtk.Builder.get_object("label_name").set_text("new text")
The task of the togglebutton is to clear the label widget display and deactivate the button which can be reactivated on the next click.
The status of togglebuttons can be retrieved by the get_active()
function returning True or False.
Depending on the widget the corresponding signal function expects at least one parameter
def on_t_button_toggled(self,widget): if widget.get_active(): #do something else: #do something different
Listings
Glade
<?xml version="1.0" encoding="UTF-8"?> <!-- Generated with glade 3.20.0 --> <interface> <requires lib="gtk+" version="3.20"/> <object class="GtkImage" id="image1"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="stock">gtk-dialog-error</property> </object> <object class="GtkApplicationWindow" id="window"> <property name="width_request">500</property> <property name="can_focus">False</property> <property name="title" translatable="yes">Titel</property> <property name="resizable">False</property> <signal name="destroy" handler="on_window_destroy" swapped="no"/> <child> <object class="GtkBox"> <property name="width_request">200</property> <property name="visible">True</property> <property name="can_focus">False</property> <property name="orientation">vertical</property> <property name="homogeneous">True</property> <child> <object class="GtkLabel" id="label"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="label" translatable="yes">Label-Text kann in Glade unter "Beschriftung" eingegeben werden</property> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">0</property> </packing> </child> <child> <object class="GtkButton" id="button"> <property name="label" translatable="yes">Label-Text ändern</property> <property name="visible">True</property> <property name="can_focus">True</property> <property name="receives_default">True</property> <signal name="clicked" handler="on_button_clicked" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">1</property> </packing> </child> <child> <object class="GtkToggleButton" id="t_button"> <property name="label" translatable="yes">Oberen Button sperren</property> <property name="visible">True</property> <property name="can_focus">True</property> <property name="receives_default">True</property> <property name="image">image1</property> <property name="image_position">top</property> <property name="always_show_image">True</property> <signal name="toggled" handler="on_t_button_toggled" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">2</property> </packing> </child> </object> </child> <child> <placeholder/> </child> </object> </interface>
Python
#!/usr/bin/python # -*- coding: utf-8 -*- import random import gi gi.require_version("Gtk", "3.0") from gi.repository import Gtk class Handler: def on_window_destroy(self, *args): Gtk.main_quit() def on_button_clicked(self,widget): new_text = random.choice(x.label_texts) x.builder.get_object("label").set_text(new_text) def on_t_button_toggled(self,widget): if widget.get_active(): x.builder.get_object("label").set_text("") x.builder.get_object("button").set_sensitive(False) else: x.builder.get_object("button").set_sensitive(True) class Example: def __init__(self): self.gladefile = "02_labelbutton.glade" self.label_texts = ["The things you used to own, now they own you.", "I am Jack's complete lack of surprise. I am Jack's Broken Heart.", "On a long enough time line, the survival rate for everyone drops to zero.", "Sticking feathers up your butt does not make you a chicken!", "I am Jack's smirking revenge."] self.builder = Gtk.Builder() self.builder.add_from_file(self.gladefile) self.builder.connect_signals(Handler()) window = self.builder.get_object("window") window.show_all() def main(self): Gtk.main() x = Example() x.main()
Comments
Comments powered by Disqus