Spoilt for choice
| Anke (encarsia) | Also available in: Deutsch
Contents
Spinbutton and Combobox
Named widgets simplify input of values by providing a value list or range. Value input is normally done via mouseclicks, key input is optional.
Glade
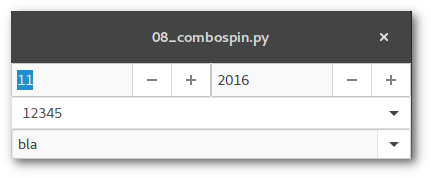
Spinbutton
In Glade some properties of spinbutton widgets can be set like the minimum/maximum/default value. It is required to tie a adjustment widget to the spinbutton. To do so select "General > Spin Button Attributes > Adjustment" and assign or create an adjustment.
Looking at the example file the two spinbuttons represent month and year dates. The month spinbutton is set to be circular, the minimum value follows after passing the maximum value. This act emits the wrapped signal which is assigned to then adjust the year date of the second spinbutton.
Combobox
There are two different combobox widgets in GTK+:
- GtkComboboxText
-
The list items of the dropdown menu are strings directly that are directly set in the Glade widget properties
- GtkCombobox
-
The list items are stored in a ListStore or TreeStore element that can hold data sets instead of a plain list. These data stores are accessible to different widgets (see the "List article").
Both widgets can include an optional entry widget which has to be activated in "General > Has Entry". By activating an internal GtkEntry widget is created. It is important to set "Common > Widget Flags > Can focus".
The first ComboboxText widget in the exampe does not possess an entry field, the user can only select an item from the list. In both widgets the changed signal is assigned to retrieve the selection.
Python
Spinbutton
The value of a spinbutton can be easily retrieved and set via get_value
and set_value
.
In the example the values for the month and year date are set with the current dates. The on_spin_m_wrapped
function changes the year date up or down according to the value set in month date.
Combobox
Combobox lists can be extended by using the append
function, p.e.
[self.builder.get_object("comboboxtext2").append(None,entry) for entry in ("foo","bar","yes","no")]
You get the selected item by calling widget.set_active_text()
which also passes the text of the optional text entry widget.
Listings
Glade
<?xml version="1.0" encoding="UTF-8"?> <!-- Generated with glade 3.20.0 --> <interface> <requires lib="gtk+" version="3.20"/> <object class="GtkAdjustment" id="adj_j"> <property name="lower">1234</property> <property name="upper">3000</property> <property name="step_increment">1</property> <property name="page_increment">10</property> </object> <object class="GtkAdjustment" id="adj_m"> <property name="lower">1</property> <property name="upper">12</property> <property name="step_increment">1</property> <property name="page_increment">10</property> </object> <object class="GtkWindow" id="window"> <property name="can_focus">False</property> <property name="default_width">400</property> <signal name="destroy" handler="on_window_destroy" swapped="no"/> <child> <object class="GtkBox"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="orientation">vertical</property> <child> <object class="GtkBox"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="homogeneous">True</property> <child> <object class="GtkSpinButton" id="spin_m"> <property name="visible">True</property> <property name="can_focus">True</property> <property name="placeholder_text" translatable="yes">Monat</property> <property name="adjustment">adj_m</property> <property name="wrap">True</property> <signal name="wrapped" handler="on_spin_m_wrapped" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">1</property> </packing> </child> <child> <object class="GtkSpinButton" id="spin_y"> <property name="visible">True</property> <property name="can_focus">True</property> <property name="adjustment">adj_j</property> <property name="climb_rate">1</property> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">2</property> </packing> </child> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">0</property> </packing> </child> <child> <object class="GtkComboBoxText" id="comboboxtext1"> <property name="visible">True</property> <property name="can_focus">False</property> <items> <item id="<Geben Sie die Kennung ein>" translatable="yes">eins</item> <item translatable="yes">zwei</item> <item translatable="yes">12345</item> <item translatable="yes">mehr Listenblabla</item> </items> <signal name="changed" handler="on_comboboxtext1_changed" swapped="no"/> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">2</property> </packing> </child> <child> <object class="GtkComboBoxText" id="comboboxtext2"> <property name="visible">True</property> <property name="can_focus">False</property> <property name="has_entry">True</property> <signal name="changed" handler="on_comboboxtext2_changed" swapped="no"/> <child internal-child="entry"> <object class="GtkEntry" id="entry"> <property name="can_focus">True</property> </object> </child> </object> <packing> <property name="expand">False</property> <property name="fill">True</property> <property name="position">3</property> </packing> </child> </object> </child> <child type="titlebar"> <placeholder/> </child> </object> </interface>
Python
#!/usr/bin/python # -*- coding: utf-8 -*- import datetime import gi gi.require_version("Gtk", "3.0") from gi.repository import Gtk class Handler: def on_window_destroy(self, *args): Gtk.main_quit() def on_spin_m_wrapped(self, widget): if widget.get_value() == 1: x.spin_y.set_value(x.spin_y.get_value() + 1) else: x.spin_y.set_value(x.spin_y.get_value() - 1) def on_comboboxtext1_changed(self, widget): print("Auswahl ComboBox 1:", widget.get_active_text()) def on_comboboxtext2_changed(self, widget): print("Auswahl ComboBox 2:", widget.get_active_text()) class Example: def __init__(self): self.builder = Gtk.Builder() self.builder.add_from_file("08_combospin.glade") self.builder.connect_signals(Handler()) #set current values for month/year self.builder.get_object("spin_m").set_value(datetime.datetime.now().month) self.spin_y = self.builder.get_object("spin_y") self.spin_y.set_value(datetime.datetime.now().year) #set combobox list values [self.builder.get_object("comboboxtext2").append(None,entry) for entry in ("bla", "blubb", "ja", "nein")] window = self.builder.get_object("window") window.show_all() def main(self): Gtk.main() x = Example() x.main()
Comments
Comments powered by Disqus